Rainbow in Python is not a function. It is simply creating a rainbow of colors for different purposes. We can use various methods to create a rainbow in Python. It all depends on your requirements and needs, you can use any of the following methods to create a rainbow in Python. In this short article, we will be using turtle and matplotlib modules to create a rainbow in Python.
Create a Rainbow in Python
As you know the rainbow is a combination of seven different colors where the first color is red and the last color is violet.
In this short article, we will create a rainbow using the following different methods:
- Rainbow in Python using the turtle module
- Rainbow Python using matplotlib module
Let us start to build a rainbow in Python using these two methods.
Rainbow Using Turtle in Python
Turtle is a built-in module in Python that provides a drawing screen and pen(turtle) to draw various shapes. Similar to physical drawing, in the Turtle module we have to move the pen to draw different shapes. Various functions are available that help move the pen in different directions.
Now let us create a rainbow in Python using the turtle module and then we will explain the code
# Import turtle package
import turtle
# Creating a turtle screen object
sc = turtle.Screen()
# Creating a turtle object(pen)
pen = turtle.Turtle()
# Defining a method to form a semicircle
def semi_circle(col, rad, val):
# Set the fill color of the semicircle
pen.color(col)
# Draw a circle
pen.circle(rad, -180)
# Move the turtle to air
pen.up()
# Move the turtle to a given position
pen.setpos(val, 0)
# Move the turtle to the ground
pen.down()
pen.right(180)
# Set the colors for drawing
col = ['violet', 'indigo', 'blue',
'green', 'yellow', 'orange', 'red']
# Setup the screen features
sc.setup(600, 600)
# Set the screen color to black
sc.bgcolor('black')
# Setup the turtle features
pen.right(90)
pen.width(10)
pen.speed(7)
# Loop to draw 7 semicircles
for i in range(7):
semi_circle(col[i], 10*(
i + 8), -10*(i + 1))
# Hide the turtle
pen.hideturtle()
Output:
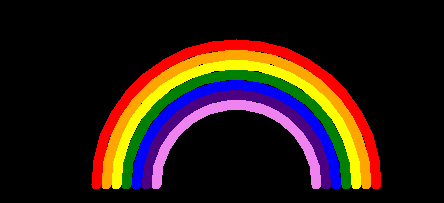
In the example above, the semi_circle function takes three parameters including color, radius, and value. This function uses the turtle (pen) to set the color of the semi-circle with a given radius.
Please also check: Stock Price Predictions
First, the turtle set up the screen including the speed and width. We have defined a loop for 7 colors so that each loop will create a different color:
Creating Rainbow in Python Using Matplotlib
Matplotlib module has many valuable functions to create different types of plots. Although there is no function to create a rainbow we can use the matplotlib module to create a rainbow shape. Let us first create a rainbow in Python using matplotlib module and then we will explain the code.
# importing the module
import matplotlib.pyplot as plt
# creating subplots
fig, ax = plt.subplots()
plt.axis("equal")
# fiing the axis of the rainbow
ax.set(xlim=(10, 0), ylim=(10, -10))
# using for loop to create rainbow color
for i in range(0, 7):
rainbow = '#FF0000', '#FF7F00','#FFFF00', '#00FF00', '#0000FF', '#4B0082', '#9400D3'
c = plt.Circle((0, 0), 7-i, fill=True, color=rainbow[i])
ax.add_artist(c)
plt.show()
Output:
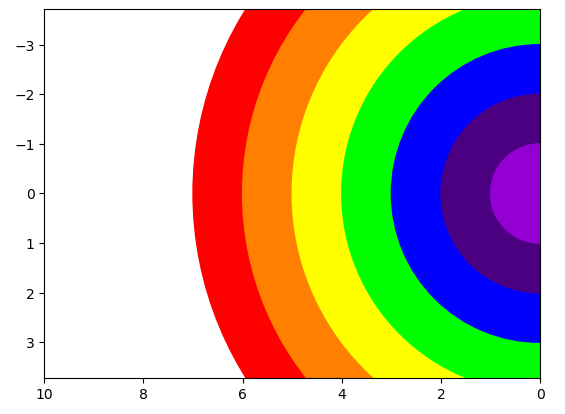
We have created a rainbow in Python using matplotlib module. First, we imported the module and then defined the axis of the plot. Then we used for loop to create a rainbow with 7 different colors:
We can also use rainbow colors as indicators in the matplotlib module. For example, we may want different scattered plots to have a rainbow color indicator. Here is the code for it:
# importing the modules
import matplotlib.pyplot as plt
import numpy as np
# Create an array of evenly spaced values between 0 and 1
x = np.linspace(0, 1, 256)
# Create a rainbow colormap with 256 colors
cmap = plt.cm.rainbow
# Plot the rainbow colormap
plt.imshow(x.reshape(1, 256), cmap=cmap, interpolation='nearest')
plt.colorbar()
plt.show()
Output:
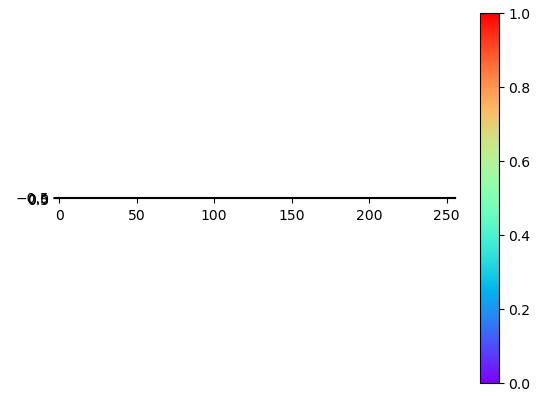
As you can see, there is a rainbow indicator.
Summary
In this article, we learned how we can create a rainbow in Python using various modules. We create a rainbow using turtle and matplotlib modules.